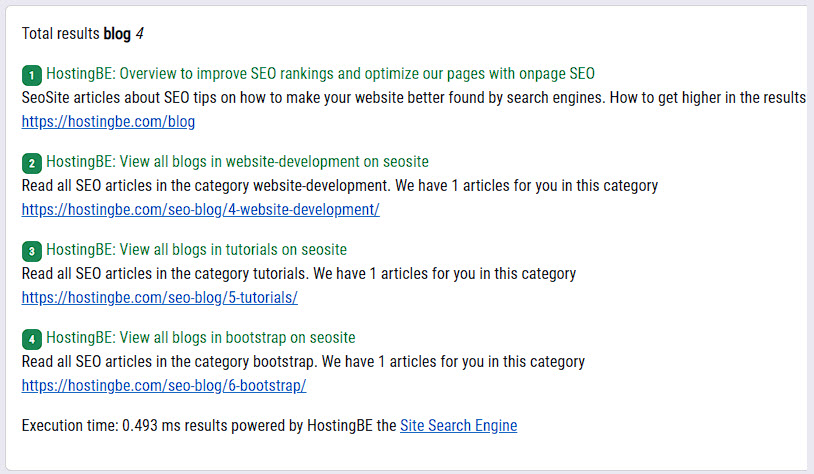
Create website search engine in php
in PhpCreate website search engine in PHP Why a search engine on your website? What requirements mus..
15-01-2023 0 reactiesIf visitors do not find the information they are looking for within a few seconds, they will move on to the next website (your competitor). A good site earch engine on your website gives the visitor the results they are looking for within milliseconds. This way you can positively influence the visitor experience on your website!
With most websites, the search functionality is the neglected child of the website. For example, wildcards are used to search a database on multiple columns. The results are then not sorted based on relevance to the search term.
A website search engine must meet various requirements such as:
First of all, you must have created an account at HostingBE and have added your website in the dashboard so that it is indexed. If your website has been indexed you will be able to retrieve the results. To be able to show these results you need 2 API tokens, namely username and password, which you can obtain in the dashboard under API settings. Also don't forget to enter your IP address which connects to the site search engine.
We first create a PHP class that connects to the API of the site search engine. We provide the search terms, which website is being searched for and the IP address of the visitor for the purpose of statistics. I deliberately keep it simple, but you can of course extend the PHP class with other functions afterwards. First we create the PHP class called SiteSearch.php
<?php
/*
* PHP class SiteSearch to connect to the API of HostingBE
*/
Class SiteSearch {
}
?>
We have now created a PHP class called SiteSearch and added some comments so we know what this PHP class does.
In this class we use the Guzzle/Client package which is an open source package that allows you to easily make http and https requests that are required to connect to the REST API. We install the Guzzle package via the command line:
composer require guzzlehttp/guzzle:^7.0
To use the Guzzle package we need to add it to our PHP class. We do this by placing the line use Guzzle/Client at the top of the script.
Furthermore, we create a constructor function in which we create the new client so that it is available in the entire PHP class. You can think of this client object as your browser requesting a new page every time.
We have to configure this new customer, first of all we provide the url. Second, the path and then we set the timeout and we want to handle the error message from http itself, hence the http_errors on false.
<?php
require(__DIR__. '/../../vendor/autoload.php');
use GuzzleHttp\Client;
/*
* PHP class SiteSearch to connect to the API of HostingBE
*/
class SiteSearch {
protected $settings = array();
protected $client;
public function __construct(array $settings) {
$this->settings = $settings;
$this->client = new Client(['base_uri' => $this->settings['url'] . $this->settings['path'],
'timeout' => 2.0,'http_errors' => false]);
}
To test whether the API connection works, we will send the ping command. We can process this ping command in our PHP class by creating a ping function that looks like this.
/**
* execute the ping command
*/
public function ping() {
$res = $this->client->request('GET','ping');
$body = $res->getBody();
return json_decode($body->getContents());
}
We create a function ping, we use the http GET method to send the ping to the API. The answer is in the $res object. Then we request the body of the response from the API and return it as an array to the script. The script we create below.
To use this ping function we create another PHP file called search.php In this file we indicate that we want to use the PHP class SiteSearch and also that we want to send the command ping, which then looks like this:
<?php
require 'SiteSearch.php';
use SiteSearch;
$username = "your API username";
$password = "your API password";
$search = new SiteSearch(['url' => 'https://api.hostingbe.com', 'path' => '/api/']);
$reply = $search->ping();
print_r($reply);
?>
If you call search.php in your browser, you will get the answer below.
stdClass Object ( [code] => 200 [status] => success [message] => Ping received, pong! )
We now have a connection to the Site Search API. In order to retrieve search results for your website, we must log in. We do this by extending our SiteSearch PHP class with a login function. This function then looks like the PHP code below.
/**
* execute the login command with username and password
*/
public function login($username, $password) {
$res = $this->client->request('POST','login',['form_params' => [
'username' => $username,
'password' => $password]
]);
$body = $res->getBody();
$data = json_decode($body->getContents());
if (($data->code == 200) && ($data->data->token)) {
$this->settoken($data->data->token);
}
return $data;
}
When calling this function, we pass along the username and password. Then we make a POST request to the API with the command login and additional information the username and password. We process the response and check if the status we get back is a status 200 code and if it includes a token that we need to use with every command we send to the API.
Finally, we call the function settoken which puts the token into a string in the PHP class SiteSearch so that we can continue to use it with other functions in the class.
The settoken function looks like below:
protected function settoken($token) {
$this->token = $token;
}
Now we can modify our PHP search.php file and add the login functionality when we run it we get the following result
stdClass Object ( [code] => 200 [status] => success [message] => Successfully retrieved a token for
QvOX9ff6okwRgZHVZAJmLwtdycX1fuIm [data] => stdClass Object ( [token] => eyJ0eXA..shortened..iOiwFo [expires] => 1673792470 ) )
The Status is 200, the command was executed successfully and we got a token which is valid for 2 hours.
We can now send a ping and login to the API. Now we will retrieve the search results with the obtained token. This function in the SiteSearch class looks like below:
public function search($q, $website,$ip = null,$referer = null) {
$res = $this->client->request('POST','search',['headers' => ['Authorization' => 'Bearer ' . $this->gettoken()],'form_params' => ['q' => $q, 'website' => $website,'ip' => $ip,'referer' => $referer]]);
return $this->handlereply($res);
}
With the search function we pass along the search term (q) we are looking for, the website for which we are searching without https or http. The ip address and the referer for the statistics. The results are shown in JSON by the API and we decode them into an array.
Now we can add this search function to our search.php file. Look below for the code.
$reply = $search->search('search engine','hostingbe.com',$_SERVER['REMOTE_ADDR'],$_SERVER['HTTP_REFERER']);
print_r($reply);
In response you get the result of the command and the search results in order of relevance.
We can now display these results on the website. For example, via a foreach loop that looks like the code below.
foreach ($reply->data->results as $result) {
print "<h1>".$result->title."</h1>";
print "<p>".$result->description."</p>";
print "<a href=".$result->url.">".$result->url."</a>";
}
We have now created a PHP search engine for your website which displays the results in order of relevance and we can also view the search statistics in the control panel.
We can now extend the search.php with, for example, breaking results into pages of more than 10. We can also add highlights to the results, which I will explain in my next post.
You can download the 2 files we have created here.
Published 2023-01-15T17:01:28 by Constan van Suchtelen van de Haereno comments on this article, or your comment is not yet approved!
Create website search engine in PHP Why a search engine on your website? What requirements mus..
15-01-2023 0 reactiesMulti step bootstrap responsive form Today we are going to make a responsive multi step form with bootstrap 5 and jquery. The multi step form consists o..
15-10-2022 0 reacties2022 © HostingBe.com CMS versie 2.2.2